win32_gui 1.0.5
win32_gui: ^1.0.5 copied to clipboard
Win32 API GUI in Object-Oriented style with some helpers. Uses package `win32` and `dart:ffi`.
win32_gui #
Win32 API GUI in Object-Oriented style with some helpers. Uses package win32 and dart:ffi.
Screenshot #
Example screenshot:
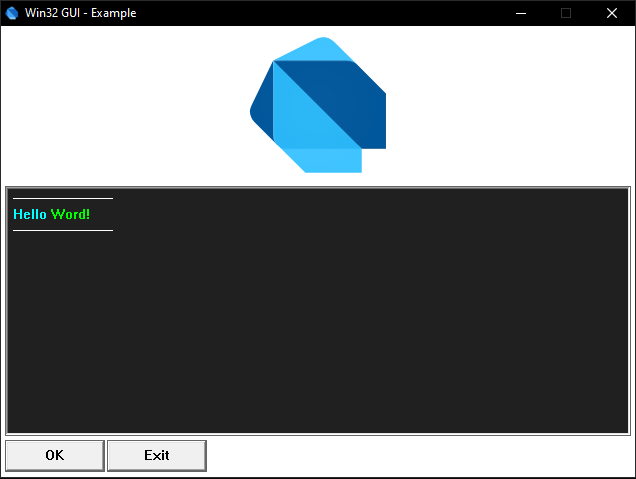
Usage #
Here's a simple Hello World window:
import 'dart:io';
import 'package:win32_gui/win32_gui.dart';
Future<void> main() async {
var mainWindow = MainWindow(
width: 640,
height: 480,
);
print('-- mainWindow.ensureLoaded...');
await mainWindow.ensureLoaded();
print('-- mainWindow.show...');
mainWindow.show();
mainWindow.onDestroy.listen((window) {
print('-- Window Destroyed> $window');
exit(0);
});
print('-- Window.runMessageLoopAsync...');
await Window.runMessageLoopAsync();
}
class MainWindow extends Window {
// Declare the main window custom class:
static final mainWindowClass = WindowClass.custom(
className: 'mainWindow',
windowProc: Pointer.fromFunction<WindowProc>(mainWindowProc, 0),
bgColor: RGB(255, 255, 255),
useDarkMode: true,
titleColor: RGB(32, 32, 32),
);
// Redirect to default implementation [WindowClass.windowProcDefault].
static int mainWindowProc(int hwnd, int uMsg, int wParam, int lParam) =>
WindowClass.windowProcDefault(
hwnd, uMsg, wParam, lParam, mainWindowClass);
MainWindow({super.width, super.height})
: super(
windowName: 'Win32 GUI - Example',
windowClass: mainWindowClass,
windowStyles: WS_MINIMIZEBOX | WS_SYSMENU,
) ;
late final String imageDartLogoPath;
late final String iconDartLogoPath;
@override
Future<void> load() async {
imageDartLogoPath = await Window.resolveFilePath(
'package:win32_gui/resources/dart-logo.bmp');
iconDartLogoPath = await Window.resolveFilePath(
'package:win32_gui/resources/dart-icon.ico');
}
@override
void build(int hwnd, int hdc) {
super.build(hwnd, hdc);
SetTextColor(hdc, RGB(255, 255, 255));
SetBkColor(hdc, RGB(96, 96, 96));
// Some extra build...
}
@override
void repaint(int hwnd, int hdc) {
super.repaint(hwnd, hdc);
setIcon(iconDartLogoPath);
var imgW = 143;
var imgH = 139;
var hBitmap = loadImageCached(imageDartLogoPath, imgW, imgH);
final x = (dimensionWidth - imgW) ~/ 2;
final y = 10;
drawImage(hdc, hBitmap, x, y, imgW, imgH);
}
}
Features and bugs #
Please file feature requests and bugs at the issue tracker.
Contribution #
Your assistance and involvement within the open-source community is not only valued but vital. Here's how you can contribute:
- Found an issue?
- Please fill a bug report with details.
- Wish a feature?
- Open a feature request with use cases.
- Are you using and liking the project?
- Advocate for the project: craft an article, share a post, or offer a donation.
- Are you a developer?
- Fix a bug and send a pull request.
- Implement a new feature.
- Improve the Unit Tests.
- Already offered your support?
- Sincere gratitude from myself, fellow contributors, and all beneficiaries of this project!
By donating one hour of your time, you can make a significant contribution, as others will also join in doing the same. Just be a part of it and begin with your one hour.
Author #
Graciliano M. Passos: gmpassos@GitHub.